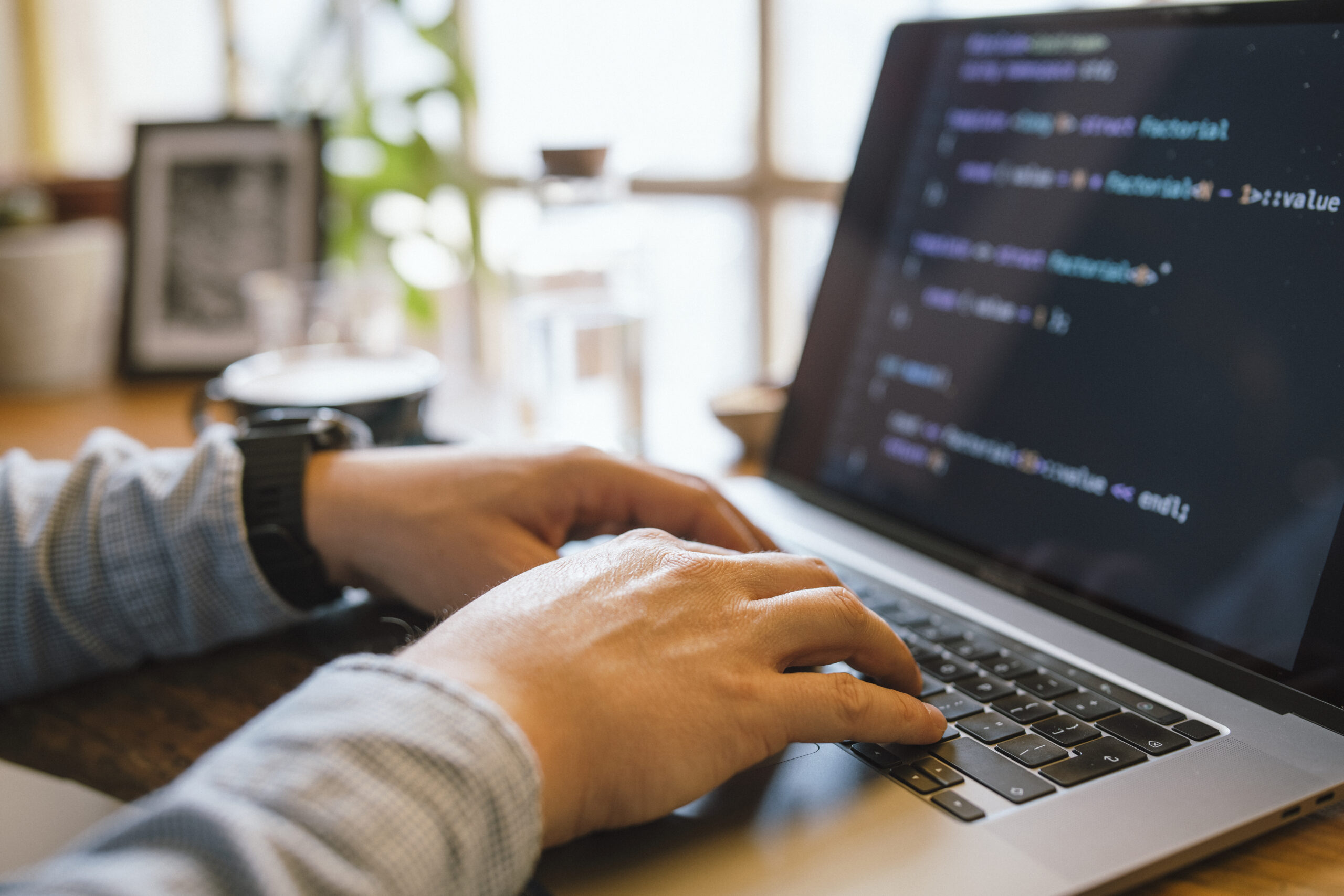
Debugging is Among the most essential — nevertheless generally missed — skills inside a developer’s toolkit. It is not almost repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can preserve hrs of stress and substantially increase your productiveness. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of advancement, knowing how to connect with it properly in the course of execution is equally significant. Present day improvement environments occur Outfitted with powerful debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code over the fly. When utilised properly, they Enable you to observe particularly how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable duties.
For backend or process-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these resources could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become comfy with Edition Regulate units like Git to understand code background, uncover the precise minute bugs had been launched, and isolate problematic alterations.
In the long run, mastering your resources means going past default settings and shortcuts — it’s about building an intimate understanding of your growth setting making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, developers have to have to make a steady atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask thoughts like: What steps resulted in The difficulty? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or mistake messages? The greater depth you have, the much easier it turns into to isolate the exact conditions beneath which the bug occurs.
As soon as you’ve collected plenty of info, endeavor to recreate the trouble in your neighborhood surroundings. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra correctly, test potential fixes safely, and communicate more Evidently with your workforce or buyers. It turns an summary complaint into a concrete obstacle — Which’s where by builders prosper.
Read through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and quickly begin building assumptions. But deeper during the mistake stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common events (like successful get started-ups), Alert for prospective problems that don’t crack the applying, ERROR for actual complications, and Deadly once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Deal with vital functions, state variations, enter/output values, and demanding conclusion factors in your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you may lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not simply a complex task—it's a type of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start off by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a clear picture of what’s going on.
Future, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations just lately been created towards the codebase? Has this issue happened in advance of beneath equivalent situations? The goal should be to slim down prospects and determine opportunity culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed natural environment. Should you suspect a specific purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay near attention to smaller specifics. Bugs often cover in the minimum expected destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may perhaps conceal the real trouble, only for it to resurface afterwards.
Finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for long term difficulties and assist Some others comprehend your reasoning.
By wondering like a detective, builders can sharpen their analytical abilities, solution difficulties methodically, and come to be more effective at uncovering hidden challenges in complicated programs.
Generate Tests
Composing checks is one of the most effective solutions to enhance your debugging capabilities and Over-all development efficiency. Exams not merely support capture bugs early but also serve as a security Web that gives you self-assurance when generating improvements towards your codebase. A well-tested application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a specific piece of logic is working as envisioned. Any time a take a look at fails, you quickly know the place to search, substantially lowering the time used debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear just after Beforehand currently being set.
Next, combine integration assessments and finish-to-end tests into your workflow. These enable be certain that different parts of your software operate with each other effortlessly. They’re notably helpful for catching bugs that occur in advanced techniques with numerous factors or providers interacting. If something breaks, your assessments can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on fixing the bug and observe your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your monitor for several hours, trying Answer right after Remedy. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential issues when the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a brand new layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is huge. It would make you a far more efficient, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you here do.